Citadin Lv.7
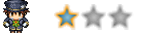
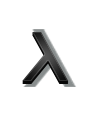
Inscrit le : 07/09/2011 Messages : 186
![[VX] Teleportation Empty](https://2img.net/i/fa/empty.gif) | Sujet: [VX] Teleportation Sam 25 Fév 2012 - 0:39 | |
| - Molok sur la palais du Making a écrit:
-
- Code:
-
#============================================================================== # Téléportation Gausienne & autre # Ajoute des effets de téléportation (S4suk3, Merci a Joke & Fabien !) #============================================================================== # ** Gausian_Stuff #------------------------------------------------------------------------------ # Utilitaires de gestion des flous #==============================================================================
module Gausian_Stuff extend self #-------------------------------------------------------------------------- # * Cast Opacity #-------------------------------------------------------------------------- def opacity_to_percent(opacity) return (((opacity.to_f)/255.0)*100).to_i end #-------------------------------------------------------------------------- # * Cast Percent #-------------------------------------------------------------------------- def percent_to_opacity(percent) return (((percent.to_f)*255.0)/100).to_i end #-------------------------------------------------------------------------- # * Process blur for one #-------------------------------------------------------------------------- def make_blur(bmp_source, facteur = 1) blur_table = Array.new(9, Sprite.new) c = 0 for i in blur_table i.opacity = (c == 0) ? 255 : 31 i.bitmap = bmp_source.clone i.ox = 272 i.oy = 208 i.z = 200 + Kernel.rand(8) c += 1 end #Process blur blur_table[1].x = 272-facteur blur_table[1].y = 208 blur_table[2].x = 272-facteur blur_table[2].y = 208-facteur blur_table[3].x = 272 blur_table[3].y = 208-facteur blur_table[4].x = 272+facteur blur_table[4].y = 208-facteur blur_table[5].x = 272+facteur blur_table[5].y = 208 blur_table[6].x = 272+facteur blur_table[6].y = 208+facteur blur_table[7].x = 272 blur_table[7].y = 208+facteur blur_table[8].x = 272-facteur blur_table[8].y = 208+facteur return blur_table end #-------------------------------------------------------------------------- # * Process blur #-------------------------------------------------------------------------- def blur_screen(arg, duration = 40) list_sprite = [] for i in 0..(duration*1.5).to_i fac = (i%2 == 0) ? arg : -arg list_sprite += Gausian_Stuff.make_blur(Graphics.snap_to_bitmap, fac) Graphics.update end return Gausian_Stuff.cut(list_sprite) end #-------------------------------------------------------------------------- # * reverse Tail Head #-------------------------------------------------------------------------- def cut(l) bmp = Graphics.snap_to_bitmap for i in l i.dispose end spr = Sprite.new spr.bitmap = bmp spr.ox = 272 spr.oy = 208 spr.x = 272 spr.y = 208 spr.opacity = 255 return spr end end
#============================================================================== # ** Game_Screen #------------------------------------------------------------------------------ # This class handles screen maintenance data, such as change in color tone, # flashes, etc. It's used within the Game_Map and Game_Troop classes. #==============================================================================
class Game_Screen #-------------------------------------------------------------------------- # * Public Instance Variables #-------------------------------------------------------------------------- attr_accessor :teleport_pics #-------------------------------------------------------------------------- # * add_teleport #-------------------------------------------------------------------------- def add_teleport @teleport_pics ||= Array.new @teleport_pics << Sprite.new t = @teleport_pics.reverse return t[0] end end
#============================================================================== # ** Scene_Map #------------------------------------------------------------------------------ # This class performs the map screen processing. #==============================================================================
class Scene_Map #-------------------------------------------------------------------------- # * Instances Vars #-------------------------------------------------------------------------- attr_accessor :spriteset end
#============================================================================== # ** Game_Interpreter #------------------------------------------------------------------------------ # Modification des téléportation #==============================================================================
class Game_Interpreter #-------------------------------------------------------------------------- # * Alias #-------------------------------------------------------------------------- alias teleport_initialize initialize alias teleport_command_201 command_201 #-------------------------------------------------------------------------- # * Instances Vars #-------------------------------------------------------------------------- attr_accessor :type_teleport #-------------------------------------------------------------------------- # * Object Initialization # depth : nest depth # main : main flag #-------------------------------------------------------------------------- def initialize(depth = 0, main = false) @type_teleport = false teleport_initialize(depth, main) end #-------------------------------------------------------------------------- # * standard trans #-------------------------------------------------------------------------- def perform(map_id, x, y, direction) $game_player.reserve_transfer(map_id, x, y, direction) bitmap = Graphics.snap_to_bitmap $scene.spriteset.dispose $game_player.perform_transfer $game_map.autoplay $game_map.update $scene.spriteset = Spriteset_Map.new Input.update return bitmap end #-------------------------------------------------------------------------- # * gfx update #-------------------------------------------------------------------------- def gfx_update Graphics.update $game_map.update $game_map.screen.update $scene.spriteset.update end #-------------------------------------------------------------------------- # * Transfer Player #-------------------------------------------------------------------------- def command_201 if @type_teleport return true if $game_temp.in_battle if $game_player.transfer? or $game_message.visible return false end if @params[0] == 0 map_id = @params[1] x = @params[2] y = @params[3] direction = @params[4] else map_id = $game_variables[@params[1]] x = $game_variables[@params[2]] y = $game_variables[@params[3]] direction = @params[4] end # Téléportation Standard sans fondu if @type_teleport == :standard bitmap = perform(map_id, x, y, direction) spr = $game_map.screen.add_teleport spr.bitmap = bitmap spr.opacity = 255 $game_map.screen.update c = 255 for i in 0...40 c -= (i*2) spr.opacity = c gfx_update end spr.dispose # Téléportation Gausienne elsif @type_teleport == :gausian l = Gausian_Stuff.blur_screen(8,15) bitmap = perform(map_id, x, y, direction) spr = $game_map.screen.add_teleport spr.z = 500 spr.bitmap = bitmap spr.opacity = 255 l.dispose $game_map.screen.update c = 255 for i in 0...40 c -= (i*2) spr.opacity = c gfx_update end spr.dispose # Téléportation Instantannée elsif @type_teleport == :instant $game_player.reserve_transfer(map_id, x, y, direction) fade = (Graphics.brightness > 0) $scene.spriteset.dispose $game_player.perform_transfer $game_map.autoplay $game_map.update $scene.spriteset = Spriteset_Map.new Input.update # Téléportation normale else teleport_command_201 end else teleport_command_201 end end #-------------------------------------------------------------------------- # * Gausian Teleport #-------------------------------------------------------------------------- def teleport_gausian @type_teleport = :gausian end #-------------------------------------------------------------------------- # * Standard Teleport #-------------------------------------------------------------------------- def teleport_standard @type_teleport = :standard end #-------------------------------------------------------------------------- # * Instant teleport #-------------------------------------------------------------------------- def teleport_instant @type_teleport = :instant end #-------------------------------------------------------------------------- # * Normal #-------------------------------------------------------------------------- def teleport_normal @type_teleport = false end end Permet de modifier les transitions: appeller script avant une transition et mettre teleport_instant et la téléportation sera instantannée teleport_standard et elle sera sans le fondu noir (avec une transition) teleport_normal et elle sera normal teleport_gausian et ce sera une tentative moisie de floue (merci sasugay)
http://vimeo.com/36317914 EXEMPLES |
|
Voyageur Lv.10
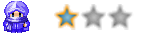
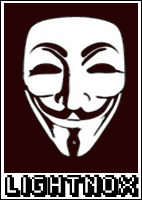
Age : 34 Inscrit le : 12/02/2009 Messages : 469
![[VX] Teleportation Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Teleportation Sam 25 Fév 2012 - 2:09 | |
| script sympa je testerais a l'occas  merci du partage  |
|
Va-nu-pieds Lv.4


Age : 27 Inscrit le : 20/02/2012 Messages : 50
![[VX] Teleportation Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Teleportation Jeu 12 Avr 2012 - 11:32 | |
| on pourrait le mettre en event commun? |
|
![[VX] Teleportation Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [VX] Teleportation ![[VX] Teleportation Icon_minitime](https://2img.net/i/fa/icon_minitime.gif) | |
| |
|