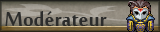
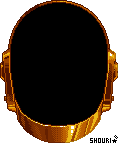
Age : 134 Inscrit le : 14/04/2009 Messages : 1321
![[Résolu] Système pour avoir 2 parallaxes Empty](https://2img.net/i/fa/empty.gif) | Sujet: Re: [Résolu] Système pour avoir 2 parallaxes Jeu 12 Mai 2011 - 16:12 | |
| Sinon, il existe un script de multi-panorama. Hum, j'ai perdu le lien d'origine, mais je l'ai encore ... Avec celui-ci, tu peux ajotuer autant de panoramas voulus. Tient : - Code:
-
#============================================================================== # (RMVX) ~ Multi-panorama ~ # de Lufia # v 1.0 #============================================================================== # Ce script permet d'afficher plusieurs panoramas / fogs en même temps durant le jeu. # Nombre maximum de panoramas : MAX_PANORAMAS = 200 # # Les images sont à placer dans le dossier Parallaxes. # Les commandes suivantes sont à utiliser en insertion de script. # # Afficher un panorama : # show_pano(id, name, z, opacity, blend_type, zoom_x, zoom_y, autoscroll_x_speed, # autoscroll_y_speed, move_x_speed, move_y_speed) # id : numéro du panorama (de 0 à MAX_PANORAMAS - 1) # name : nom du fchier dans Graphics/Parallaxes # z : coordonnée en z, si ce nombre est grand, le panorama apparaît au-dessus d'autres # éléments de la carte # opacity : opacité (de 0 à 255, nombre décimaux autorisés) # blend_type : type de transparence (0 : normale, 1 : addition, 2 : soustraction) # zoom_x : zoom en x (taille normale : 100.0) # zoom_y : zoom en y (taille normale : 100.0) # autoscroll_x_speed : vitesse de défilement horizontal # autoscroll_y_speed : vitesse de défilement vertical # move_x_speed : scrolling parallaxe en x (même vitesse que la carte : 2) # move_y_speed : scrolling parallaxe en y (même vitesse que la carte : 2) # ex : show_pano(0, "Light", 100, 50, 2, 200, 200, 0, 0, 2, 2) # # Modifier un panorama : # change_pano_properties(id, z, opacity, blend_type, zoom_x, zoom_y, autoscroll_x_speed, # autoscroll_y_speed, move_x_speed, move_y_speed, duration, wait_for_end) # duration : temps de transition (en frames) # wait_for_end : attendre la fin de la transition ? (oui : true / non : false) # ex : change_pano_properties(0, 20, 255, 0, 100, 100, 0, 15, 2, 2, 20, true) # # Modifier le ton d'un panorama : # change_pano_tone(id, red, green, blue, gray, duration, wait_for_end) # red : ajustement du rouge (de -255 à 255) # green : ajustement du vert (de -255 à 255) # blue : ajustement du bleu (de -255 à 255) # gray : ajustement du gris (de -255 à 255) # ex : change_pano_tone(0, 100, -100, 0, 35, 15, false) # # Modifier la couleur d'un panorama : # change_pano_color(id, red, green, blue, alpha, duration, wait_for_end) # red : valeur du rouge (de 0 à 255) # green : valeur du vert (de 0 à 255) # blue : valeur du bleu (de 0 à 255) # alpha : alpha blending (de 0 à 255) # ex : change_pano_color(0, 255, 100, 0, 0, 0, false) # # Effacer un panorama : # hide_pano(args) # args : ids des panoramas # ex : hide_pano(1, 3, 12) # #============================================================================== # Merci à Zeus81 pour ses précieux conseils. # Enjoy ! #==============================================================================
#============================================================================== # ** Game_Panorama #------------------------------------------------------------------------------ # This class handles panoramas. This class is used within the Game_Map class. #============================================================================== class Game_Panorama
#-------------------------------------------------------------------------- # * Public Instance Variables #-------------------------------------------------------------------------- attr_reader :id attr_accessor :name attr_accessor :z attr_accessor :opacity attr_accessor :blend_type attr_accessor :zoom_x attr_accessor :zoom_y attr_accessor :color attr_accessor :tone attr_accessor :autoscroll_x_speed attr_accessor :autoscroll_y_speed attr_accessor :move_x_speed attr_accessor :move_y_speed #-------------------------------------------------------------------------- # * Object initialization # id : panorama number #-------------------------------------------------------------------------- def initialize(id) @id = id @name = "" @z = 0 @opacity = 255.0 @target_opacity = 255.0 @blend_type = 0 @zoom_x = 100.0 @target_zoom_x = 100.0 @zoom_y = 100.0 @target_zoom_y = 100.0 @color = Color.new(0, 0, 0, 0) @target_color = Color.new(0, 0, 0, 0) @tone = Tone.new(0, 0, 0, 0) @target_tone = Tone.new(0, 0, 0, 0) @autoscroll_x_speed = 0 @autoscroll_y_speed = 0 @move_x_speed = 0.0 @move_y_speed = 0.0 @duration = 0 @tone_duration = 0 @color_duration = 0 end #-------------------------------------------------------------------------- # * Show panorama # name : filename # z : z coordinate # opacity : sprite opacity # blend_type : blend type # zoom_x : x axis zoom # zoom_y : y axis zoom # autoscroll_x_speed : x axis autoscroll speed # autoscroll_y_speed : y axis autoscroll speed # move_x_speed : x axis scroll speed # move_y_speed : y axis scroll speed #-------------------------------------------------------------------------- def show(name, z, opacity, blend_type, zoom_x, zoom_y, autoscroll_x_speed, autoscroll_y_speed, move_x_speed, move_y_speed) @name = name @z = z @opacity = opacity.to_f @target_opacity = opacity @blend_type = blend_type @zoom_x = zoom_x.to_f @target_zoom_x = @zoom_x @zoom_y = zoom_y.to_f @target_zoom_y = @zoom_y @color = Color.new(0, 0, 0, 0) @target_color = @color.clone @tone = Tone.new(0, 0, 0, 0) @target_tone = @tone.clone @autoscroll_x_speed = autoscroll_x_speed @autoscroll_y_speed = autoscroll_y_speed @move_x_speed = move_x_speed.to_f @move_y_speed = move_y_speed.to_f end #-------------------------------------------------------------------------- # * Erase panorama #-------------------------------------------------------------------------- def hide @name = "" end #-------------------------------------------------------------------------- # * Change panorama properties # z : z coordinate # zoom_x : x axis zoom # zoom_y : y axis zoom # blend_type : blend type # opacity : sprite opacity # duration : transition time #-------------------------------------------------------------------------- def change_opacity(z, zoom_x, zoom_y, opacity, blend_type, autoscroll_x_speed, autoscroll_y_speed, move_x_speed, move_y_speed, duration) @z = z @blend_type = blend_type @autoscroll_x_speed = autoscroll_x_speed @autoscroll_y_speed = autoscroll_y_speed @move_x_speed = move_x_speed.to_f @move_y_speed = move_y_speed.to_f @target_zoom_x = zoom_x.to_f @target_zoom_y = zoom_y.to_f @target_opacity = opacity.to_f @duration = duration if duration == 0 @zoom_x = @target_zoom_x @zoom_y = @target_zoom_y @opacity = @target_opacity end end #-------------------------------------------------------------------------- # * Change panorama tone # tone : new tone (Tone object) # duration : transition time #-------------------------------------------------------------------------- def change_tone(tone, duration) @target_tone = tone.clone @tone_duration = duration if duration == 0 @tone = @target_tone.clone end end #-------------------------------------------------------------------------- # * Change panorama color # color : new color (Color object) # duration : transition time #-------------------------------------------------------------------------- def change_color(color, duration) @target_color = color.clone @color_duration = duration if duration == 0 @color = @target_color.clone end end #-------------------------------------------------------------------------- # * Frame update #-------------------------------------------------------------------------- def update if @duration > 0 d = @duration @zoom_x = (@zoom_x * (d-1) + @target_zoom_x) / d @zoom_y = (@zoom_y * (d-1) + @target_zoom_y) / d @opacity = (@opacity * (d-1) + @target_opacity) / d @duration -= 1 end if @tone_duration > 0 d = @tone_duration @tone.red = (@tone.red * (d-1) + @target_tone.red) / d @tone.green = (@tone.green * (d-1) + @target_tone.green) / d @tone.blue = (@tone.blue * (d-1) + @target_tone.blue) / d @tone.gray = (@tone.gray * (d-1) + @target_tone.gray) / d @tone_duration -= 1 end if @color_duration > 0 d = @color_duration @color.red = (@color.red * (d-1) + @target_color.red) / d @color.green = (@color.green * (d-1) + @target_color.green) / d @color.blue = (@color.blue * (d-1) + @target_color.blue) / d @color.alpha = (@color.alpha * (d-1) + @target_color.alpha) / d @color_duration -= 1 end end end
#============================================================================== # ** Game_Map #------------------------------------------------------------------------------ # This class handles maps. It includes scrolling and passage determination # functions. The instance of this class is referenced by $game_map. #============================================================================== class Game_Map
#-------------------------------------------------------------------------- # * Public Instance Variables #-------------------------------------------------------------------------- attr_accessor :panoramas #-------------------------------------------------------------------------- # * Object initialization #-------------------------------------------------------------------------- alias lufia_multipano_initialize initialize def initialize @panoramas = [] for i in 0...MAX_PANORAMAS @panoramas.push(Game_Panorama.new(i)) end lufia_multipano_initialize end #-------------------------------------------------------------------------- # * Frame Update #-------------------------------------------------------------------------- alias lufia_multipano_update update def update @panoramas.each { |i| i.update } lufia_multipano_update end end
#============================================================================== # ** Sprite_Panorama #------------------------------------------------------------------------------ # This plane is used to display panoramas. It observes a instance of the # Game_Panorama class and automatically changes plane properties. #============================================================================== class Sprite_Panorama < Plane #-------------------------------------------------------------------------- # * Object initialization # pano : panorama (Game_Panorama) #-------------------------------------------------------------------------- def initialize(pano) super() @pano = pano @name = "" update end #-------------------------------------------------------------------------- # * Frame update #-------------------------------------------------------------------------- def update if @pano.name != @name @name = @pano.name if @name.empty? self.bitmap = nil self.visible = false else self.bitmap = Cache.parallax(@name) @width_x_8, @height_x_8 = self.bitmap.width * 8, self.bitmap.height * 8 @scroll_x = @scroll_y = 0 self.visible = true end end unless @name.empty? self.z = @pano.z self.opacity = @pano.opacity self.blend_type = @pano.blend_type self.zoom_x = @pano.zoom_x / 100.0 self.zoom_y = @pano.zoom_y / 100.0 self.color = @pano.color self.tone = @pano.tone @scroll_x = (@scroll_x + @pano.autoscroll_x_speed) % @width_x_8 @scroll_y = (@scroll_y + @pano.autoscroll_y_speed) % @height_x_8 self.ox = (@scroll_x + $game_map.display_x * @pano.move_x_speed) / 8 self.oy = (@scroll_y + $game_map.display_y * @pano.move_y_speed) / 8 end end end
#============================================================================== # ** Spriteset_Map #------------------------------------------------------------------------------ # This class brings together map screen sprites, tilemaps, etc. It's used # within the Scene_Map class. #============================================================================== class Spriteset_Map #-------------------------------------------------------------------------- # * Create parallax #-------------------------------------------------------------------------- alias lufia_multipano_create_parallax create_parallax def create_parallax @sprites_panoramas = [] for i in 0...MAX_PANORAMAS @sprites_panoramas.push(Sprite_Panorama.new($game_map.panoramas[i])) end lufia_multipano_create_parallax end #-------------------------------------------------------------------------- # * Dispose parallax #-------------------------------------------------------------------------- alias lufia_multipano_dispose_parallax dispose_parallax def dispose_parallax lufia_multipano_dispose_parallax @sprites_panoramas.each { |i| i.dispose if i != nil } end
#-------------------------------------------------------------------------- # * Update parallax #-------------------------------------------------------------------------- alias lufia_multipano_update_parallax update_parallax def update_parallax lufia_multipano_update_parallax for pano in @sprites_panoramas pano.update end end end
#============================================================================== # ** Game_Interpreter #------------------------------------------------------------------------------ # An interpreter for executing event commands. This class is used within the # Game_Map, Game_Troop, and Game_Event classes. #============================================================================== class Game_Interpreter #-------------------------------------------------------------------------- # * Show panorama # id : panorama number # name : filemane # z : z coordinate # opacity : sprite opacity # blend_type : blend type # zoom_x : x axis zoom # zoom_y : y axis zoom # autoscroll_x_speed : x axis autoscroll speed # autoscroll_y_speed : y axis autoscroll speed # move_x_speed : x axis scroll speed # move_y_speed : y axis scroll speed #-------------------------------------------------------------------------- def show_pano(id, name, z, opacity, blend_type, zoom_x, zoom_y, autoscroll_x_speed, autoscroll_y_speed, move_x_speed, move_y_speed) $game_map.panoramas[id].show(name, z, opacity, blend_type, zoom_x, zoom_y, autoscroll_x_speed, autoscroll_y_speed, move_x_speed, move_y_speed) return true end #-------------------------------------------------------------------------- # * Erase panorama # args : panorama numbers #-------------------------------------------------------------------------- def hide_pano(*args) args.each { |id| $game_map.panoramas[id].hide } return true end #-------------------------------------------------------------------------- # * Change panorama properties # id : panorama number # z : z coordinate # zoom_x : x axis zoom # zoom_y : y axis zoom # opacity : sprite opacity # blend_type : blend type # autoscroll_x_speed : x axis autoscroll speed # autoscroll_y_speed : y axis autoscroll speed # move_x_speed : x axis scroll speed # move_y_speed : y axis scroll speed # duration : transition time # wait_for_end : wait for end of transition? #-------------------------------------------------------------------------- def change_pano_properties(id, z, opacity, blend_type, zoom_x, zoom_y, autoscroll_x_speed, autoscroll_y_speed, move_x_speed, move_y_speed, duration, wait_for_end) $game_map.panoramas[id].change_opacity(z, zoom_x, zoom_y, opacity, blend_type, autoscroll_x_speed, autoscroll_y_speed, move_x_speed, move_y_speed, duration) @wait_count = duration if wait_for_end return true end #-------------------------------------------------------------------------- # * Change panorama tone # id : panorama number # red : red value (-255 - 255) # green : green value (-255 - 255) # blue : blue value (-255 - 255) # gray : grayscale value (-255 - 255) # duration : transition time # wait_for_end : wait for end of transition? #-------------------------------------------------------------------------- def change_pano_tone(id, red, green, blue, gray, duration, wait_for_end) $game_map.panoramas[id].change_tone(Tone.new(red, green, blue, gray), duration) @wait_count = duration if wait_for_end return true end #-------------------------------------------------------------------------- # * Change panorama color # id : panorama number # red : red value (0 - 255) # green : green value (0 - 255) # blue : blue value (0 - 255) # alpha : alpha blending (0 - 255) # duration : transition time # wait_for_end : wait for end of transition? #-------------------------------------------------------------------------- def change_pano_color(id, red, green, blue, alpha, duration, wait_for_end) $game_map.panoramas[id].change_color(Color.new(red, green, blue, alpha), duration) @wait_count = duration if wait_for_end return true end end
|
|